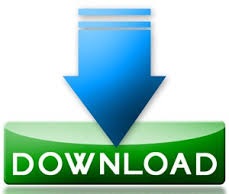
The resultCode value shows whether the request is ok or canceled.
In the Source Activity java class, override onActivityResult(int requestCode, int resultCode, Intent dataIntent) method, the requestCode is just the user-defined integer number that is passed in above startActivityForResult method, use the requestCode to check which startActivityForResult request can use the returned dataIntent object if there are multiple places invoke the startActivityForResult method in the Source Activity. The first parameter can be _OK or RESULT_CANCELED, the second parameter is the intent object. #USING INTENT ANDROID STUDIO ANDROID#
Call setResult(RESULT_OK, intent) method in the Target Activity to set the returned intent in android os.
intent.putExtra("message_return", "This data is returned when user click button in target activity.")
Call above intent object’s putExtra(String key, Object data) method to set the returned result data in the intent object. Do not need to provide constructor parameters at this time.
To return data back to Source Activity from the Target Activity, you should create an instance of class in the Target Activity. startActivityForResult(intent, REQUEST_CODE_1) The first parameter is the intent object, the second parameter is a user-defined integer number that is used to check whether the returned intent object is related to this request. If you want to get the response data from the Target Activity, now you should call startActivityForResult(Intent intent, int requestCode) method in the Source Activity, this method will pass the intent object to android os and wait for the response from the Target Activity. intent.putExtra("message", "This message comes from PassingDataSourceActivity's second button") Store the passed data in the above intent object by invoking its putExtra(String key, Object data) method, the key is a message key, the data is the passed data value. Intent intent = new Intent(PassingDataSourceActivity.this, PassingDataTargetActivity.class) Create a New Explicit or Implicit class’s instance in the Source Activity. String message = intent.getStringExtra("message") 3. The key should be the same as step 2 when invoking the intent object’s putExtra method. In the Target Activity, call the above intent object’s getStringExtra(String key) to get Source Activity passed data. In the Target Activity, call getIntent() method to get the Source Activity sent intent object. Invoke Source Activity object’s startActivity(intent) method to pass the intent object to the android os.
intent.putExtra("message", "This message comes from PassingDataSourceActivity's first button") Invoke the above intent object’s putExtra(String key, Object data) method to store the data that will pass to Target Activity in it.Create an instance of class, pass the Source Activity object ( who sent the intent object ) and the Target Activity class ( who can receive the intent object ) to the Intent class constructor.Pass Data Between Activities Use Intent Object. If you click the second button in the Source Activity, it will pass data to the Target Activity also, and when you click the return button or click the android Back menu at the bottom in the Target Activity, the Source Activity can get the Target Activity returned response result data and display the result text in the Source Activity.If you click the first button in the Source Activity, it will pass data to the Target Activity, and when you click the return button in the Target Activity, the Source Activity can not get the response data from Target Activity.
The Target Activity contains one button ( PASS RESULT DATA BACK TO SOURCE ACTIVITY ).The Source Activity contains two buttons ( PASS DATA TO NEXT ACTIVITY and PASS DATA TO NEXT ACTIVITY AND GET RESULT BACK ).There are two activities in this example, a Source Activity, and a Target Activity.